In this short post, I’ll share a simple script to modify the YML files. You can also use the script in the CI/CD pipelines, an example for Azure DevOps is below.
To read the YML file we will use powershell-yaml
module, which you can install and active like this:
1 2 |
Install-Module -Name powershell-yaml Import-Module powershell-yaml |
Here is our sample YML file that we will use in our little demo:
1 2 3 4 5 6 7 |
header: "This is header" data: param1: "Awesome parameter" param2: "will be replaced" footer: title: "Footer Title" body: "will be replaced" |
And here is a PowerShell script, that will modify the above YML file. It contains some comments, so you can read through.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
# Arguments that get passed to the script when running it param ( [Parameter(Position=1)] $Param2, [Parameter(Position=2)] $FooterBody ) # Install and import the `powershell-yaml` module # Install module has a -Force -Verbose -Scope CurrentUser arguments which might be necessary in your CI/CD environment to install the module Install-Module -Name powershell-yaml -Force -Verbose -Scope CurrentUser Import-Module powershell-yaml # LoadYml function that will read YML file and deserialize it function LoadYml { param ( $FileName ) # Load file content to a string array containing all YML file lines [string[]]$fileContent = Get-Content $FileName $content = '' # Convert a string array to a string foreach ($line in $fileContent) { $content = $content + "`n" + $line } # Deserialize a string to the PowerShell object $yml = ConvertFrom-YAML $content # return the object Write-Output $yml } # WriteYml function that writes the YML content to a file function WriteYml { param ( $FileName, $Content ) #Serialize a PowerShell object to string $result = ConvertTo-YAML $Content #write to a file Set-Content -Path $FileName -Value $result } # Loading yml, setting new values and writing it back to disk $yml = LoadYml "sample.yml" $yml.data.param2 = $Param2 $yml.footer.body = $FooterBody WriteYml "sample.yml" $yml |
Save both files to the same folder. Now when you run .\script.ps1 -Param2 "Param value" -FooterBody "Footer value"
your sample YML file is modified:
1 2 3 4 5 6 7 |
data: param1: Awesome parameter param2: Param value footer: body: Footer value title: Footer Title header: This is header |
Now let’s include the process above in the Azure DevOps pipeline. I want to set the Param2
and FooterBody
as variables and then pass them to the script. I will not go into detail about how to set the whole pipeline, but I’ll show you the important bits. To continue, save the script above in the code repository so you will be able to reference it from PowerShell task.
Step 1: Set the pipeline variables

Step 2: Add PowerShell task to the pipeline

Step 3: Set PowerShell script task
Now click on the PowerShell task and set it up.
- Set
Type
toFile Path
. - Set
Script Path
to the script in your code repository. - In the
Arguments
field type-Param2 $(Param2) -FooterBody $(FooterBody)
which will inject the pipeline variables to the script. - Depending on where your YML files are in the code repository structure, you also need to specify the context or
Working directory
for the script.
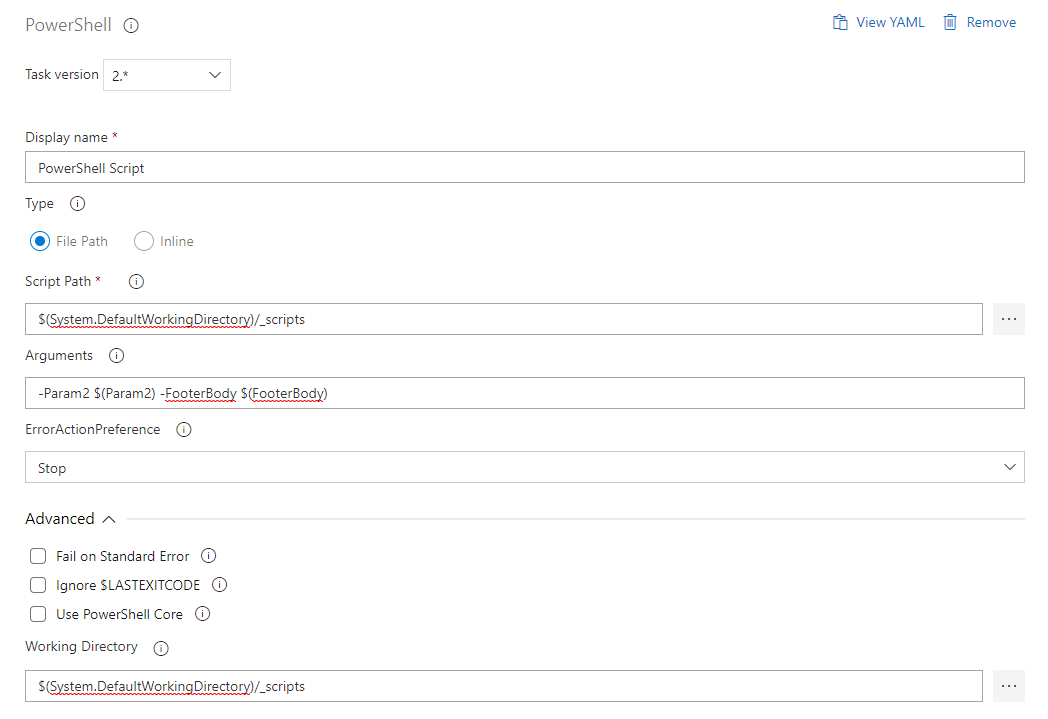
And that’s it.
I know, I know, the script can be more general and dynamic. For example, we could specify a YML file full path as the argument for the script, but for the sake of this demonstration that is fine. Leave your comments down below :).
TA-DA!
Leave a Reply
You must belogged in to post a comment.